React Hooks Deep Dive
A comprehensive guide to React Hooks. Learn how to leverage hooks to build powerful and reusable components in React.

Mario Yonan
7 mins
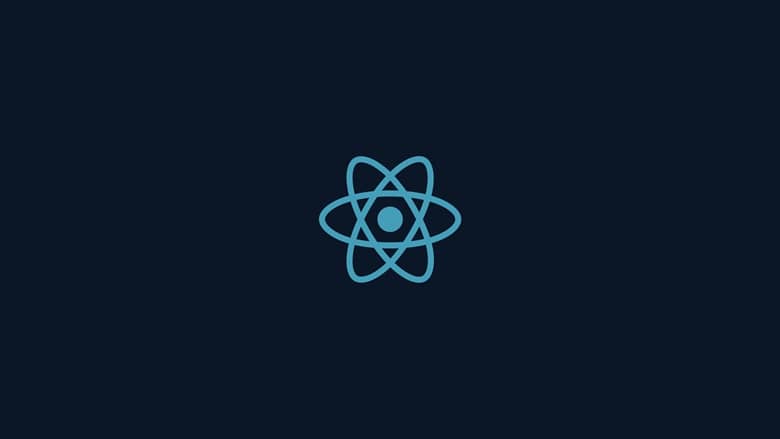
A comprehensive guide to React Hooks. Learn how to leverage hooks to build powerful and reusable components in React.
Mario Yonan
7 mins
Enjoyed this article? Share it with a friend on Twitter! Got questions, feedback or want to reach out directly? Feel free to send me a DM and I'll get back to you as soon as I can.
Have an amazing day!
Subscribe to my newsletter
Coming soon!
React Hooks are a feature introduced in React 16.8 that allows you to use state and other React features without writing a class. They provide a more concise and readable way to manage state and side effects in functional components.
Before delving into specific examples and use cases, it’s essential to understand the rules of using React hooks to ensure code consistency and prevent unexpected behavior. Adhering to these rules will help maintain the integrity of your components and ensure that hooks are used correctly:
Adhering to these rules ensures that your components are predictable, maintainable, and compatible with future updates to React.
The useState Hook is a function provided by React that allows functional components to manage state. It returns a stateful value and a function to update that value, similar to this.state and this.setState in class components.
The useState Hook takes an initial state as its argument and returns an array with two elements: the current state value and a function to update the state.
const [state, setState] = useState(initialState);
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
};
To update the state with the useState Hook, you call the state updater function returned by useState, passing it the new state value.
setCount(count + 1);
You can also use functional updates with the useState Hook, especially when the new state value depends on the previous state.
setCount((prevCount) => prevCount + 1);
The useEffect Hook is used in functional components to perform side effects. It allows you to perform operations such as data fetching, subscriptions, or manually changing the DOM after the component has rendered.
The useEffect Hook accepts two arguments: a function containing the side effect, and an optional array of dependencies. The function will run after every render unless specified otherwise.
useEffect(() => {
// Side effect code here
}, [dependencies]);
import React, { useState, useEffect } from 'react';
const ExampleComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
// Update the document title using the browser API
document.title = `You clicked ${count} times`;
}, [count]); // Only re-run the effect if count changes
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
};
You can return a cleanup function from the useEffect Hook, which will be called before the component unmounts or before the effect runs again.
useEffect(() => {
const subscription = subscribe();
return () => {
// Cleanup function here
subscription.unsubscribe();
};
}, []);
By providing a dependency array, you can specify when the effect should be re-run. If the array is empty, the effect only runs once after the initial render.
The useContext Hook provides a way to consume context within functional components in React. Context allows you to pass data through the component tree without having to pass props down manually at every level.
The useContext Hook accepts a context object (created with React.createContext) and returns the current context value for that context.
const value = useContext(MyContext);
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
function ThemedButton() {
const theme = useContext(ThemeContext);
return <button style={{ background: theme }}>I am styled by theme context!</button>;
}
function App() {
return (
<ThemeContext.Provider value="dark">
<ThemedButton />
</ThemeContext.Provider>
);
}
Custom Hooks are JavaScript functions that utilize React’s Hook API. They allow you to extract reusable logic from components, making your code more modular and easier to maintain.
To create a custom hook, simply define a JavaScript function prefixed with use. Inside the function, you can use built-in Hooks or other custom Hooks.
import { useState, useEffect } from 'react';
function useCustomHook(initialValue) {
const [value, setValue] = useState(initialValue);
useEffect(() => {
// Optional effect logic...
}, [value]);
return value;
}
import { useState } from 'react';
function useCounter(initialCount, step) {
const [count, setCount] = useState(initialCount);
const increment = () => setCount(count + step);
const decrement = () => setCount(count - step);
return { count, increment, decrement };
}
You can use custom hooks just like built-in hooks within functional components.
function CounterComponent() {
const { count, increment, decrement } = useCounter(0, 1);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
In this deep dive into React hooks, we’ve explored the fundamental hooks provided by React and learned how they revolutionize state management and side effects handling in functional components.
From useState for managing component state to useEffect for handling side effects, useContext for global state management, and custom hooks for code reusability, React hooks offer a powerful and intuitive way to build modern web applications.
By mastering React hooks, you can streamline your development workflow, write cleaner and more maintainable code, and unlock new possibilities for building dynamic and responsive user interfaces.