Testing React Applications
Master React app testing with Jest, React Testing Library, and Cypress. Learn testing importance, types, and best practices.

Mario Yonan
15 mins
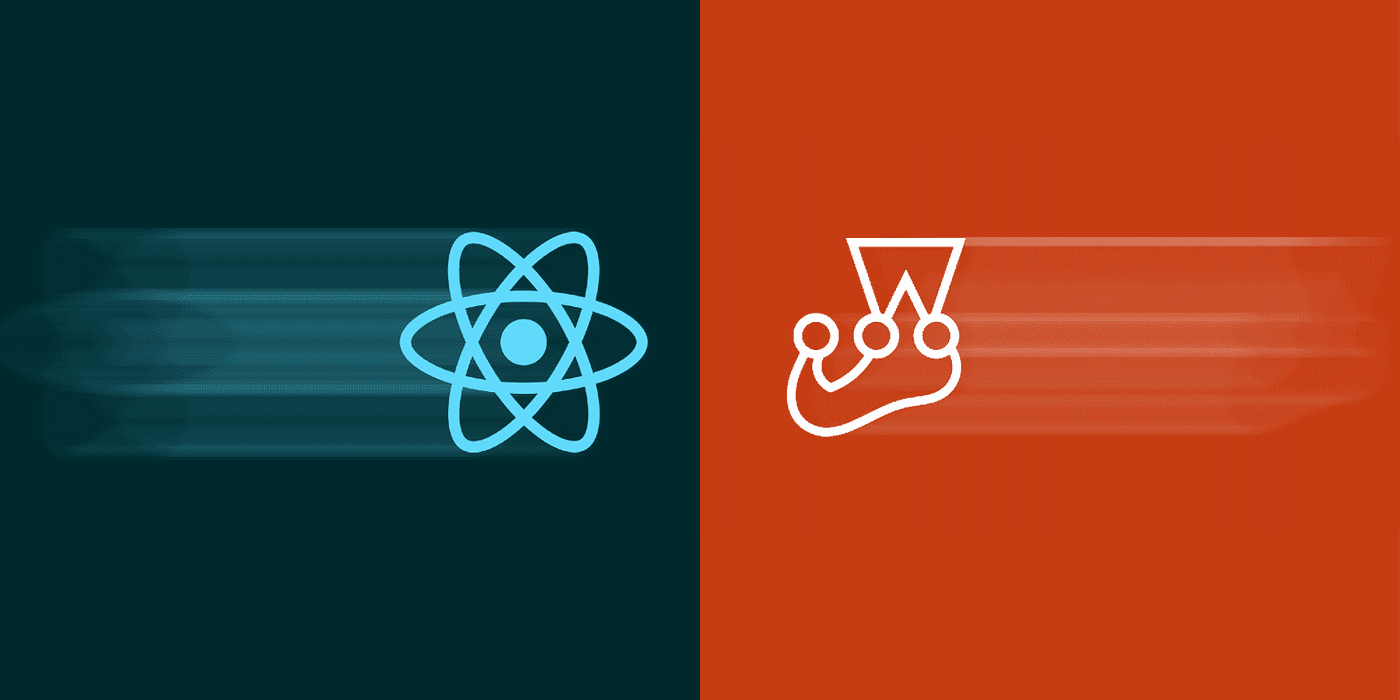
Master React app testing with Jest, React Testing Library, and Cypress. Learn testing importance, types, and best practices.
Mario Yonan
15 mins
Enjoyed this article? Share it with a friend on Twitter! Got questions, feedback or want to reach out directly? Feel free to send me a DM and I'll get back to you as soon as I can.
Have an amazing day!
Newsletter
Get personal updates and readings on topics like tech, productivity, and more!
👋 Hello everyone!
Let’s dive into an essential aspect of developing robust React applications – testing. While it’s a well-known practice in the developer community, effectively testing React apps can still be challenging.
In this article, we’ll explore various aspects of testing React applications. We’ll cover the basics, like why testing is important and the different types of tests you should write. Then we’ll get our hands dirty with some practical examples using popular tools like Jest, React Testing Library, and Cypress.
By the end of this article, you’ll have a solid understanding of how to set up a robust testing strategy for your React apps, making your development process smoother and your applications more reliable.
Let’s get started!
Testing is a critical component of software development, and for React applications, it’s no different. Here’s why testing your React apps is essential:
Understanding the importance of testing helps in appreciating the effort put into writing and maintaining tests. It’s not just about finding bugs but also about building a reliable and maintainable codebase.
Understanding the basics of testing is crucial before diving into the specifics of testing React applications. Here are some key concepts and strategies:
Understanding these fundamentals will provide a strong foundation as we move into writing specific types of tests for React applications.
Unit testing focuses on verifying the functionality of individual components in isolation. Jest and React Testing Library are commonly used together to write unit tests for React applications.
First, you need to install Jest and React Testing Library. If you haven’t already, you can add them to your project using npm or yarn:
npm install --save-dev jest @testing-library/react @testing-library/jest-dom
# or
yarn add --dev jest @testing-library/react @testing-library/jest-dom
Next, create a setupTests.js file in your src directory to configure Jest and React Testing Library:
// src/setupTests.js
import '@testing-library/jest-dom';
Ensure your package.json includes the following configuration for Jest:
{
"scripts": {
"test": "jest"
},
"jest": {
"setupFilesAfterEnv": ["<rootDir>/src/setupTests.js"],
"testEnvironment": "jsdom"
}
}
Let’s start with a simple example. Suppose you have a Button component:
// src/components/Button.js
import React from 'react';
const Button = ({ label, onClick }) => (
<button onClick={onClick}>{label}</button>
);
export default Button;
Now, let’s write a unit test for this component:
// src/components/Button.test.js
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import Button from './Button';
test('renders the button with the correct label', () => {
const { getByText } = render(<Button label="Click me" />);
expect(getByText('Click me')).toBeInTheDocument();
});
test('calls the onClick handler when clicked', () => {
const handleClick = jest.fn();
const { getByText } = render(<Button label="Click me" onClick={handleClick} />);
fireEvent.click(getByText('Click me'));
expect(handleClick).toHaveBeenCalledTimes(1);
});
React Testing Library encourages testing components in a way that resembles how users interact with them. Here are a few more examples:
Testing State Management: Suppose you have a Counter component that increments a counter when a button is clicked:
// src/components/Counter.js
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
Now, let’s write a unit test for this component:
// src/components/Counter.test.js
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import Counter from './Counter';
test('increments the counter when the button is clicked', () => {
const { getByText } = render(<Counter />);
const button = getByText('Increment');
fireEvent.click(button);
expect(getByText('Count: 1')).toBeInTheDocument();
});
Testing Props: Ensure that components render correctly based on different props:
// src/components/Greeting.js
import React from 'react';
const Greeting = ({ name }) => <h1>Hello, {name}!</h1>;
export default Greeting;
// src/components/Greeting.test.js
import React from 'react';
import { render } from '@testing-library/react';
import Greeting from './Greeting';
test('renders the correct greeting message', () => {
const { getByText } = render(<Greeting name="Alice" />);
expect(getByText('Hello, Alice!')).toBeInTheDocument();
});
Integration testing focuses on verifying the interactions between different parts of your application to ensure they work together correctly. This type of testing is crucial for React applications, where components often interact with each other and external services.
To start writing integration tests, you’ll use the same tools as for unit testing, such as Jest and React Testing Library. However, you’ll focus on testing how multiple components interact with each other.
Here’s how to set up a basic integration test:
Install necessary libraries: Make sure you have Jest and React Testing Library installed.
npm install --save-dev jest @testing-library/react @testing-library/jest-dom
# or
yarn add --dev jest @testing-library/react @testing-library/jest-dom
Configure Jest for integration testing: Ensure your Jest setup can handle integration tests, especially if you’re mocking APIs or using other external services.
Let’s consider an example where you have a UserList component that fetches and displays a list of users. This component interacts with an API and another User component.
// src/components/User.js
import React from 'react';
const User = ({ name }) => <li>{name}</li>;
export default User;
// src/components/UserList.js
import React from 'react';
import User from './User';
const UserList = ({users}) => {
return (
<ul>
{users.map(user => (
<User key={user.id} name={user.name} />
))}
</ul>
);
};
export default UserList;
Integration test
// src/components/UserList.test.js
import React from 'react';
import { render, screen, waitFor } from '@testing-library/react';
import UserList from './UserList';
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
test('fetches and displays users', async () => {
render(<UserList users=["Alice", "Bob"] />);
expect(screen.getByText('Alice')).toBeInTheDocument();
expect(screen.getByText('Bob')).toBeInTheDocument();
});
End-to-end (E2E) testing is a critical part of ensuring your React application works as expected from the user’s perspective. Cypress is a popular tool for E2E testing due to its developer-friendly features and powerful capabilities.
End-to-end testing simulates real user interactions with your application, testing the entire workflow from start to finish. This type of testing helps ensure that all components of your application work together as intended, providing a seamless experience for users.
To get started with Cypress, follow these steps:
npm install cypress --save-dev
# or
yarn add cypress --dev
npx cypress open
{
"baseUrl": "http://localhost:3000"
}
Let’s write a simple E2E test to verify the login functionality of a React application:
Create a Test File: Create a new test file in the cypress/integration folder.
// cypress/integration/login.spec.js
describe('Login', () => {
it('should log in the user successfully', () => {
cy.visit('/login');
cy.get('input[name="username"]').type('testuser');
cy.get('input[name="password"]').type('password123');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/dashboard');
cy.get('.welcome-message').should('contain', 'Welcome, testuser');
});
});
2.Run the Test: Run the test using the Cypress Test Runner.
npx cypress open
Cypress makes it easy to run and debug tests with its robust set of features:
By using Cypress for end-to-end testing, you can ensure that your React application delivers a reliable and user-friendly experience.
Test-Driven Development (TDD) is a software development methodology where tests are written before the actual code. This approach ensures that the code meets the specified requirements and helps maintain high code quality.
TDD is based on a simple cycle of writing tests, writing code, and refactoring. Here are the core principles:
Let’s walk through a simple TDD example for a React component:
Step 1: Write a Test
Suppose we want to create a Counter component. We’ll start by writing a test:
// src/components/Counter.test.js
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import Counter from './Counter';
test('increments counter when button is clicked', () => {
const { getByText } = render(<Counter />);
const button = getByText('Increment');
const counter = getByText('Count: 0');
fireEvent.click(button);
expect(counter).toHaveTextContent('Count: 1');
});
Step 2: Run the Test
Run the test to ensure it fails, indicating that the functionality is not yet implemented:
npm test
# or
yarn test
Step 3: Write the Code
Write the minimal code to pass the test:
// src/components/Counter.js
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
Step 4: Run the Test Again
Run the test again to ensure it passes with the new code:
npm test
# or
yarn test
Step 5: Refactor
Refactor the code to improve its structure and readability while ensuring the test still passes. In this simple example, the initial implementation is already quite clean, so minimal refactoring is needed.
By following the TDD workflow, you can ensure that your code is thoroughly tested and meets the desired requirements from the outset.
Mocking is an essential technique in testing that allows you to isolate the component or function under test by simulating the behavior of dependencies. This helps ensure that tests are focused and reliable.
Jest provides powerful mocking capabilities that allow you to create mocks for functions, modules, and even entire libraries.
Suppose you have a utility function that performs a complex calculation, and you want to mock it in your tests:
// src/utils/calculate.js
export const calculate = (a, b) => a + b;
// src/components/Calculator.js
import React from 'react';
import { calculate } from '../utils/calculate';
const Calculator = ({ a, b }) => {
const result = calculate(a, b);
return <div>Result: {result}</div>;
};
export default Calculator;
// src/components/Calculator.test.js
import React from 'react';
import { render } from '@testing-library/react';
import Calculator from './Calculator';
import * as calculateModule from '../utils/calculate';
jest.mock('../utils/calculate');
test('renders the result of the calculation', () => {
calculateModule.calculate.mockImplementation(() => 42);
const { getByText } = render(<Calculator a={1} b={2} />);
expect(getByText('Result: 42')).toBeInTheDocument();
});
By mocking the calculate function, you can control its behavior and test different scenarios without relying on the actual implementation.
Writing effective tests for your React applications involves following best practices that ensure your tests are maintainable, reliable, and efficient. Here are some tips to help you achieve that:
Continuous Integration and Continuous Deployment (CI/CD) are essential practices for modern software development. They automate the process of integrating code changes, running tests, and deploying applications, ensuring that your software is always in a deployable state.
CI/CD pipelines automate the testing process, allowing you to:
Several CI/CD platforms are popular in the development community for their ease of use and powerful features. Here are a few:
Once your CI/CD pipeline is set up, every code change will trigger the workflow, running your tests and providing feedback. You can monitor the status of your workflows directly in the GitHub Actions tab of your repository.
By integrating CI/CD into your development workflow, you can maintain high code quality, reduce the risk of introducing bugs, and ensure that new features are delivered to users quickly and reliably.
Implementing a robust testing strategy is crucial for delivering high-quality, reliable software. By integrating tools like Jest, React Testing Library, and Cypress into your workflow, you can catch bugs early, improve code quality, and ensure a smooth user experience. Continuous Integration and Deployment (CI/CD) further enhance this process by automating tests and deployments, maintaining the stability of your application.
Remember, testing is not just about finding bugs—it’s about ensuring that your code behaves as expected and continues to do so as it evolves. By prioritizing testing in your development workflow, you can build more reliable and maintainable React applications.